Types of Data Members:
Java Class is a collection of data members and functions. Any java program may contain two types of data members. They are;
1. Instance or non-static data members
2. Static or class data members
The following table describes the difference between the two.

Types of Methods:
In java program generally we may define two types of methods apart from constructor. They are;
1. Instance or non –static methods
2. Static or class methods
The following table describes the difference between the two.
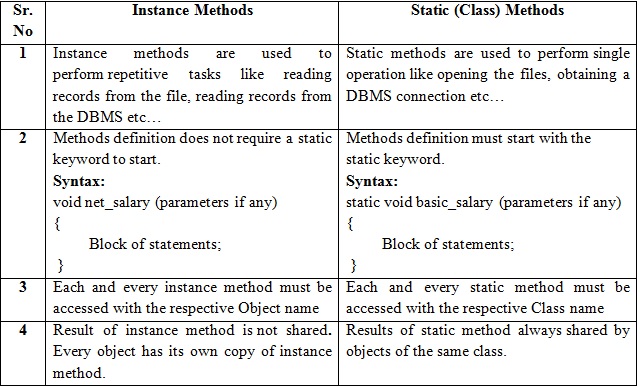
The following example named TestGVP.java demonstrates the use of different members of the java class.
Java Class is a collection of data members and functions. Any java program may contain two types of data members. They are;
1. Instance or non-static data members
2. Static or class data members
The following table describes the difference between the two.

Types of Methods:
In java program generally we may define two types of methods apart from constructor. They are;
1. Instance or non –static methods
2. Static or class methods
The following table describes the difference between the two.
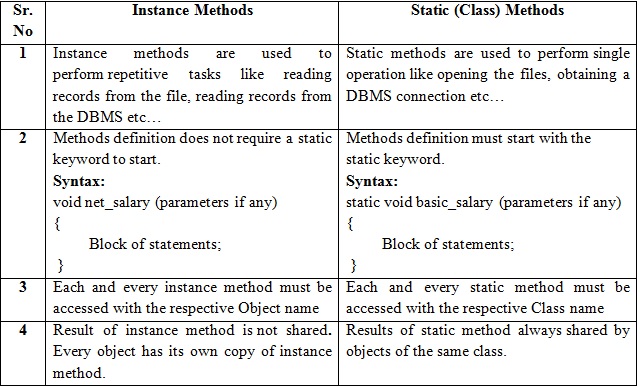
The following example named TestGVP.java demonstrates the use of different members of the java class.
// Java code to show structures and
// members of Java Program
public
class
classMember
{
// Static member
static
int
staticNum =
0
;
// Instance member
int
instanceNum;
/* below constructor increments the static
number and initialize instance number */
public
classMember(
int
i)
//Constructor method
{
instanceNum = i;
staticNum++;
}
/* The show method display the value in the staticNum and instanceNum */
public
void
show()
//instance method
{
System.out.println(
"Value of Static Number is:"
+ staticNum +
"\nValue of Instance number is:"
+ instanceNum);
}
// To find cube
public
static
int
cube()
//Static method
{
return
staticNum * staticNum * staticNum;
}
// Driver code
public
static
void
main(String args[])
{
classMember gvp1 =
new
classMember(
2
);
System.out.println(
"Value after gvp1 object creation: "
);
gvp1.show();
classMember gvp2 =
new
classMember(
4
);
System.out.println(
"Value after gvp2 object creation: "
);
gvp2.show();
// static method can be accessed by class name
int
cub=classMember.cube();
System.out.println(
"Cube of the Static number is: "
+ cub);
}
}
For output execute and get answer